本文介绍如何在Springboot中通过@Cacheable注解实现数据缓存。在每次调用添加了@Cacheable注解的方法(需要缓存功能的方法)时,Spring 会检查指定参数的指定目标方法是否已经被调用过,如果有就直接从缓存中获取方法调用后的结果,如果没有就调用方法并缓存结果后返回给用户。下次调用直接从缓存中获取。
1、添加 @EnableCaching
使用 @EnableCaching
标识在 SpringBoot 的主启动类上,开启基于注解的缓存。
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application .class, args);
}
}
2、添加
@Cacheable
在需要缓存的方法上添加
@Cacheable注解。以后查询相同的数据,直接从缓存中取,不需要调用方法。
@Cacheable(value = "areaTreeData")
public CommonResult<List<Map<String, Object>>> queryTreeData(Long pId, Long lv) {
Map<String, Object> map = new HashMap<>();
map.put("state", 1);
List<Map<String, Object>> list = getTreeData(map, pId, lv);
return new CommonResult<>(list);
}
注意:
1、返回的数据类型必须支持序列化或实现了Serializable接口,否则数据没法缓存。
2、只有直接调用该方法才能缓存,不能通过类中的其他方法来调用。
本文介绍如何在Springboot中通过@Cacheable注解实现数据缓存。在每次调用添加了@Cacheable注解的方法(需要缓存功能的方法)时,Spring 会检查指定参数的指定目标方法是否已经被调用过,如果有就直接从缓存中获取方法调用后的结果,如果没有就调用方法并缓存结果后返回给用户。下次调用直接从缓存中获取。
1、添加 @EnableCaching
使用 @EnableCaching
标识在 SpringBoot 的主启动类上,开启基于注解的缓存。
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application .class, args);
}
}
2、添加
@Cacheable
在需要缓存的方法上添加
@Cacheable注解。以后查询相同的数据,直接从缓存中取,不需要调用方法。
@Cacheable(value = "areaTreeData")
public CommonResult<List<Map<String, Object>>> queryTreeData(Long pId, Long lv) {
Map<String, Object> map = new HashMap<>();
map.put("state", 1);
List<Map<String, Object>> list = getTreeData(map, pId, lv);
return new CommonResult<>(list);
}
注意:
1、返回的数据类型必须支持序列化或实现了Serializable接口,否则数据没法缓存。
2、只有直接调用该方法才能缓存,不能通过类中的其他方法来调用。
3、常用属性说明
cacheNames/value :用来指定缓存组件的名字
key :缓存数据时使用的 key,可以用它来指定。默认是使用方法参数的值。(这个 key 你可以使用 spEL 表达式来编写)
keyGenerator :key 的生成器。 key 和 keyGenerator 二选一使用
cacheManager :可以用来指定缓存管理器。从哪个缓存管理器里面获取缓存。
condition :可以用来指定符合条件的情况下才缓存
unless :否定缓存。当 unless 指定的条件为 true ,方法的返回值就不会被缓存。当然你也可以获取到结果进行判断。(通过 #result 获取方法结果)
sync :是否使用异步模式。
4、@CacheEvict注解
@CachEvict 的作用 主要针对方法配置,能够根据一定的条件对缓存进行清空。常用属性参数如下:
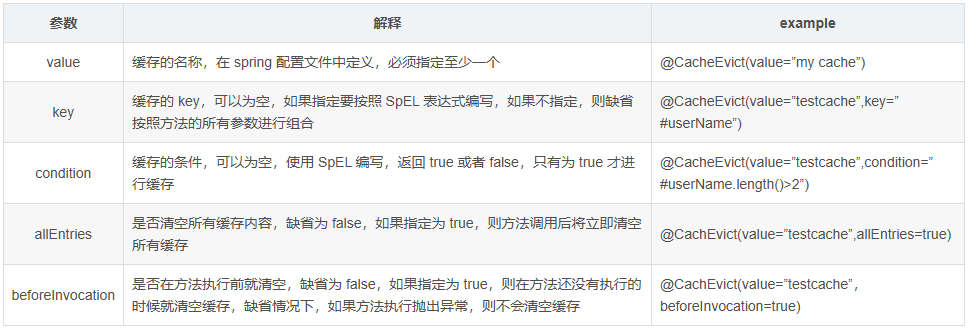
@CacheEvict(value = "areaTreeData", allEntries = true, beforeInvocation = true)
public Integer save(SysArea sysArea) {
return mapper.insertSelective(sysArea);
}